Antonio Karlsson
3D Character Artist​
3D Animator​
Technical Artist​​
Scripts
Randomizer Tool
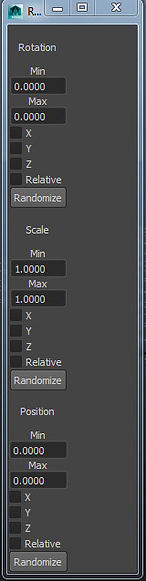
"""
Author: Antonio Karlsson
Email: antoniokarlsson85@gmail.com
"""
import maya.cmds as cmds
import random
class randomUI():
def __init__(self):
"""
UI
"""
# Give Window a name.
window_name = "myRandomGui"
window_title = "Random Generator"
# Check if window exist - if it does, then delete it.
if cmds.window(window_name, q=True, exists=True):
cmds.deleteUI(window_name)
# Create Window
my_window = cmds.window( window_name, w=10, title = window_title)
# UI Layouts - needs more work!
layout1 = cmds.rowColumnLayout( nc = 1 , parent=my_window)
layout2 = cmds.columnLayout( parent=my_window)
layout3 = cmds.rowColumnLayout( nc = 1 , parent=my_window)
layout4 = cmds.columnLayout( parent=my_window)
layout5 = cmds.rowColumnLayout( nc = 1 , parent=my_window)
layout6 = cmds.columnLayout( parent=my_window)
"""
Translation
"""
# UI Labels
cmds.text(l="", p = layout2)
cmds.text(l="Position",p=layout1)
cmds.text(l="", p = layout1)
# Adds a Float Field for the Min & Max random values.
cmds.text(l="Min",p=layout1)
self.tX_floatMin = cmds.floatField( p=layout1)
cmds.text(l="Max",p=layout1)
self.tX_floatMax = cmds.floatField( p=layout1)
# Checkboxes for each option.
self.t_X_Box = cmds.checkBox(l="X",p=layout1)
self.t_Y_Box = cmds.checkBox(l="Y",p=layout1)
self.t_Z_Box = cmds.checkBox(l="Z",p=layout1)
self.t_Relative_Box = cmds.checkBox(l="Relative",p=layout1)
# Button to randomize.
self.RandButtonTranslate = cmds.button( l="Randomize", c=self.makeRandomTrans,p=layout1)
"""
Rotation
"""
# UI Labels
cmds.text(l="", p = layout4)
cmds.text(l="Rotation",p=layout3)
cmds.text(l="", p = layout3)
# Adds a Float Field for the Min & Max random values.
cmds.text(l="Min",p=layout3)
self.rX_floatMin = cmds.floatField( p=layout3)
cmds.text(l="Max",p=layout3)
self.rX_floatMax = cmds.floatField( p=layout3)
# Checkboxes for each option.
self.r_X_Box = cmds.checkBox(l="X",p=layout3)
self.r_Y_Box = cmds.checkBox(l="Y",p=layout3)
self.r_Z_Box = cmds.checkBox(l="Z",p=layout3)
self.r_Relative_Box = cmds.checkBox(l="Relative",p=layout3)
# Button to randomize.
self.RandButtonRotate = cmds.button( l="Randomize",c=self.makeRandomRotation,p=layout3)
"""
Scale
"""
# UI Labels
cmds.text(l="", p = layout6)
cmds.text(l="Scale",p=layout5)
cmds.text(l="", p = layout5)
# Adds a Float Field for the Min & Max random values.
cmds.text(l="Min",p=layout5)
self.sX_floatMin = cmds.floatField( v=1, p=layout5)
cmds.text(l="Max",p=layout5)
self.sX_floatMax = cmds.floatField( v=1, p=layout5)
# Checkboxes for each option.
self.s_X_Box = cmds.checkBox(l="X",p=layout5)
self.s_Y_Box = cmds.checkBox(l="Y",p=layout5)
self.s_Z_Box = cmds.checkBox(l="Z",p=layout5)
self.s_Relative_Box = cmds.checkBox(l="Relative",p=layout5)
# Button to randomize.
self.RandButtonScale = cmds.button( l="Randomize",c=self.makeRandomScale ,p=layout5)
# Display Window.
cmds.showWindow(window_name)
"""
--------------------
Functions
--------------------
"""
def makeRandomTrans(self,arg):
# A List of what you currently have selected.
selection = cmds.ls(sl=True, type="transform")
# Check what number Min & Max values have.
t_Min = cmds.floatField( self.tX_floatMin, q=True, value=True)
t_Max = cmds.floatField(self.tX_floatMax, q=True, value=True)
# Check what options is True.
t_x_CheckBox = cmds.checkBox(self.t_X_Box, q=True, value=True)
t_y_CheckBox = cmds.checkBox(self.t_Y_Box, q=True, value=True)
t_z_CheckBox = cmds.checkBox(self.t_Z_Box, q=True, value=True)
t_Relative_CheckBox = cmds.checkBox(self.t_Relative_Box, q=True, value=True)
# If option is True then give a random number between Min & Max Values, if False then give the values 0,0.
for objects in selection:
if t_x_CheckBox == True:
t_Rand_X = random.uniform(t_Min,t_Max)
else:
t_Rand_X = random.uniform(0,0)
if t_y_CheckBox == True:
t_Rand_Y = random.uniform(t_Min,t_Max)
else:
t_Rand_Y = random.uniform(0,0)
if t_z_CheckBox == True:
t_Rand_Z = random.uniform(t_Min,t_Max)
else:
t_Rand_Z = random.uniform(0,0)
# If Relative option is False - make it Absolute.
if t_Relative_CheckBox == True:
cmds.xform(objects, a=False, r=True , t=(t_Rand_X,t_Rand_Y,t_Rand_Z))
else:
cmds.xform(objects, a=True, r=False , t=(t_Rand_X,t_Rand_Y,t_Rand_Z))
# Debug Helpers - prints value to script editor.
print "Min Value is",str(t_Min),'\n',"Max Value is",str(t_Max)
print "X Random Number is: ",str(t_Rand_X),'\n',"X Tick is:",t_x_CheckBox
print "Y Random Number is: ",str(t_Rand_Y),'\n',"Y Tick is:",t_y_CheckBox
print "Z Random Number is: ",str(t_Rand_Z),'\n',"Z Tick is:",t_z_CheckBox
def makeRandomRotation(self,arg):
# A List of what you currently have selected.
selection = cmds.ls(sl=True, type="transform")
# Check what number Min & Max values have.
r_Min = cmds.floatField( self.rX_floatMin, q=True, value=True)
r_Max = cmds.floatField(self.rX_floatMax, q=True, value=True)
# Check what options is True.
r_x_CheckBox = cmds.checkBox(self.r_X_Box, q=True, value=True)
r_y_CheckBox = cmds.checkBox(self.r_Y_Box, q=True, value=True)
r_z_CheckBox = cmds.checkBox(self.r_Z_Box, q=True, value=True)
r_Relative_CheckBox = cmds.checkBox(self.r_Relative_Box, q=True, value=True)
# If option is True then give a random number between Min & Max Values, if False then give the values 0,0.
for objects in selection:
if r_x_CheckBox == True:
r_Rand_X = random.uniform(r_Min,r_Max)
else:
r_Rand_X = random.uniform(0,0)
if r_y_CheckBox == True:
r_Rand_Y = random.uniform(r_Min,r_Max)
else:
r_Rand_Y = random.uniform(0,0)
if r_z_CheckBox == True:
r_Rand_Z = random.uniform(r_Min,r_Max)
else:
r_Rand_Z = random.uniform(0,0)
# If Relative option is False - make it Absolute.
if r_Relative_CheckBox == True:
cmds.xform(objects, a=False, r=True , ro=(r_Rand_X,r_Rand_Y,r_Rand_Z))
else:
cmds.xform(objects, a=True, r=False , ro=(r_Rand_X,r_Rand_Y,r_Rand_Z))
# Debug Helpers - prints value to script editor.
print "Min Value is",str(r_Min),'\n',"Max Value is",str(r_Max)
print "X Random Number is: ",str(r_Rand_X),'\n',"X Tick is:",r_x_CheckBox
print "Y Random Number is: ",str(r_Rand_Y),'\n',"Y Tick is:",r_y_CheckBox
print "Z Random Number is: ",str(r_Rand_Z),'\n',"Z Tick is:",r_z_CheckBox
def makeRandomScale(self,arg):
# A List of what you currently have selected.
selection = cmds.ls(sl=True, type="transform")
# Check what number Min & Max values have.
s_Min = cmds.floatField( self.sX_floatMin, q=True, value=True)
s_Max = cmds.floatField(self.sX_floatMax, q=True, value=True)
# Check what options is True.
s_x_CheckBox = cmds.checkBox(self.s_X_Box, q=True, value=True)
s_y_CheckBox = cmds.checkBox(self.s_Y_Box, q=True, value=True)
s_z_CheckBox = cmds.checkBox(self.s_Z_Box, q=True, value=True)
s_Relative_CheckBox = cmds.checkBox(self.s_Relative_Box, q=True, value=True)
# If option is True then give a random number between Min & Max Values, if False then give the values 1,1.
for objects in selection:
if s_x_CheckBox == True:
s_Rand_X = random.uniform(s_Min,s_Max)
else:
s_Rand_X = random.uniform(1,1)
if s_y_CheckBox == True:
s_Rand_Y = random.uniform(s_Min,s_Max)
else:
s_Rand_Y = random.uniform(1,1)
if s_z_CheckBox == True:
s_Rand_Z = random.uniform(s_Min,s_Max)
else:
s_Rand_Z = random.uniform(1,1)
# If Relative option is False - make it Absolute.
if s_Relative_CheckBox == True:
cmds.xform(objects, a=False, r=True , s=(s_Rand_X,s_Rand_Y,s_Rand_Z))
else:
cmds.xform(objects, a=True, r=False , s=(s_Rand_X,s_Rand_Y,s_Rand_Z))
# Debug Helpers - prints value to script editor.
print "Min Value is",str(s_Min),'\n',"Max Value is",str(s_Max)
print "X Random Number is: ",str(s_Rand_X),'\n',"X Tick is:",s_x_CheckBox
print "Y Random Number is: ",str(s_Rand_Y),'\n',"Y Tick is:",s_y_CheckBox
print "Z Random Number is: ",str(s_Rand_Z),'\n',"Z Tick is:",s_z_CheckBox
randomUI()